Imagine not being allowed to buy a gallon of milk because you’re color blind.
What a ridiculous scenario right!? But the reality is: Grocery stores don’t discriminate against those with disabilities…but your website (probably) does.
The reality is that there are millions and millions of people with disabilities who use the web every day. As a community, we cannot continue to treat them as an afterthought. When I say this, please take note: I’m not trying to shame anyone. When I am talking about our community, I truly mean it; it’s on all of us, including WebDevStudios, to make improvements in this regard.
If you’re not familiar, “accessibility” is the practice of writing code and creating websites that are easy to read and navigate for those who cannot view or hear in a traditional manner. This is not another article preaching the importance of accessibility. There are plenty articles focused on WHY, but even fewer that explain HOW. This will be an article on how, as I take a high-level view of the different types of accessibility concerns, showcase some code examples, and finally introduce you to some software to help you test it all out.
If you’re interested in learning why it’s important (and it is), please consider reading this fantastic article on A List Apart, check to see if it’s the law where you live, then get caught up on W3C standards, WordPress Accessibility Standards, and look at some tutorials on writing accessible code.
Note: For the remainder of this article, I may refer to “accessibility” as “a11y”.
Types of Accessibility Concerns
There are many types of disabilities, but the three big ones that pertain to the web are:
Visual Impairment
- Color blindness (Monochromacy, red-green, blue-yellow)
- Partially sighted (Can be corrected with contact lenses or glasses)
- Low vision (Cannot be corrected. Cataracts, glaucoma, macular degeneration, etc…)
- Total blindness
Physical Impairment
- Limited mobility (Those who rely on a keyboard, other hardware, or have trouble using a mouse)
Hearing Impairment
- Partial impairment (low hearing, or deaf in one ear)
- Total deafness
Visual Impairment Testing Techniques
Color blindness
Check the contrast. This the easiest way to ensure your website is viewable to those who are colorblind. You can use a tools like spurapp.com, colorable, or simply take a screenshot of your website, import into Photoshop, and then change the image to ‘grayscale’. Your text should stand out, links should be slightly different from the text, and the content sections should be well defined with visual cues such as a line (rather than backgrounds).
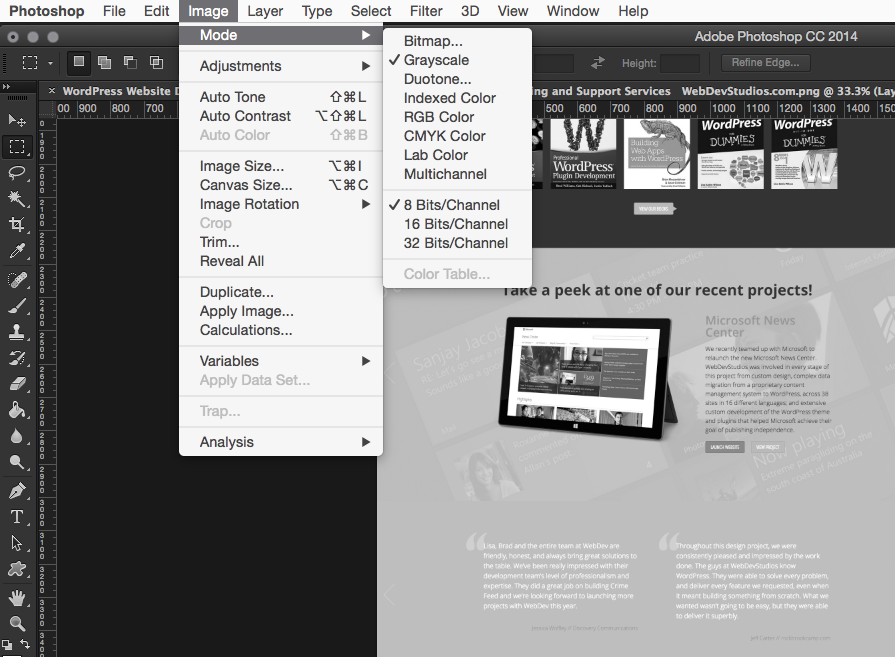
Partially sighted
View your website with enlarged text by zooming (CMD + plus or minus), as the text becomes larger does your website break? Remember NOT to use pixels when setting your font-size. Instead, use EMs or REMs.
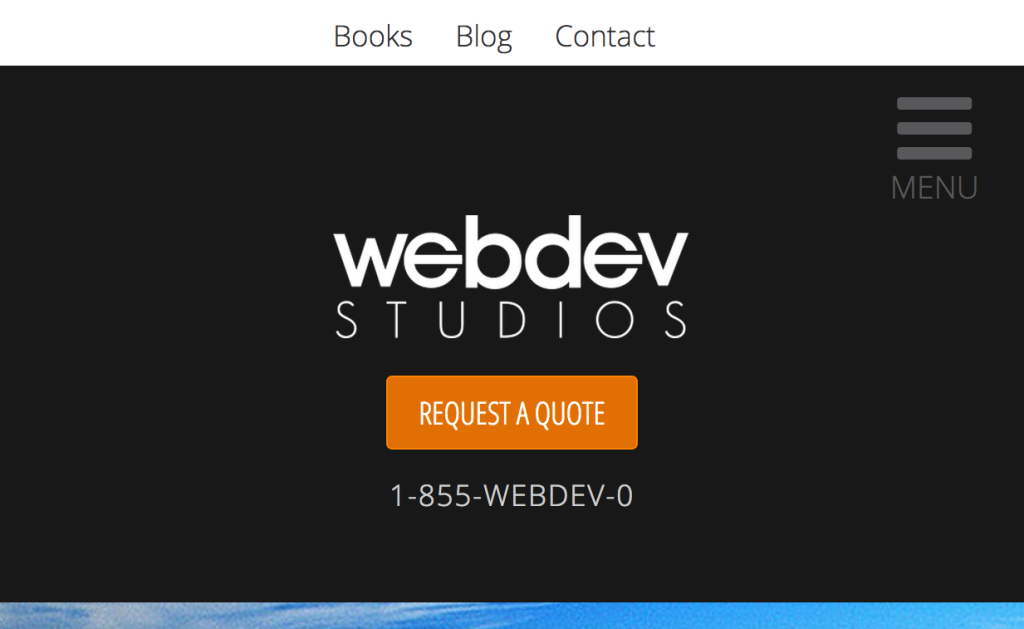
Low vision
If you’re using spurapp.com, click on the “blur” tool, or with a screenshot of your website in Photoshop, blur your website. Is your content still easy to see or read? Try adding a lot of vignetting, how does your site look now? This is how it would appear to someone with say cataracts or glaucoma.
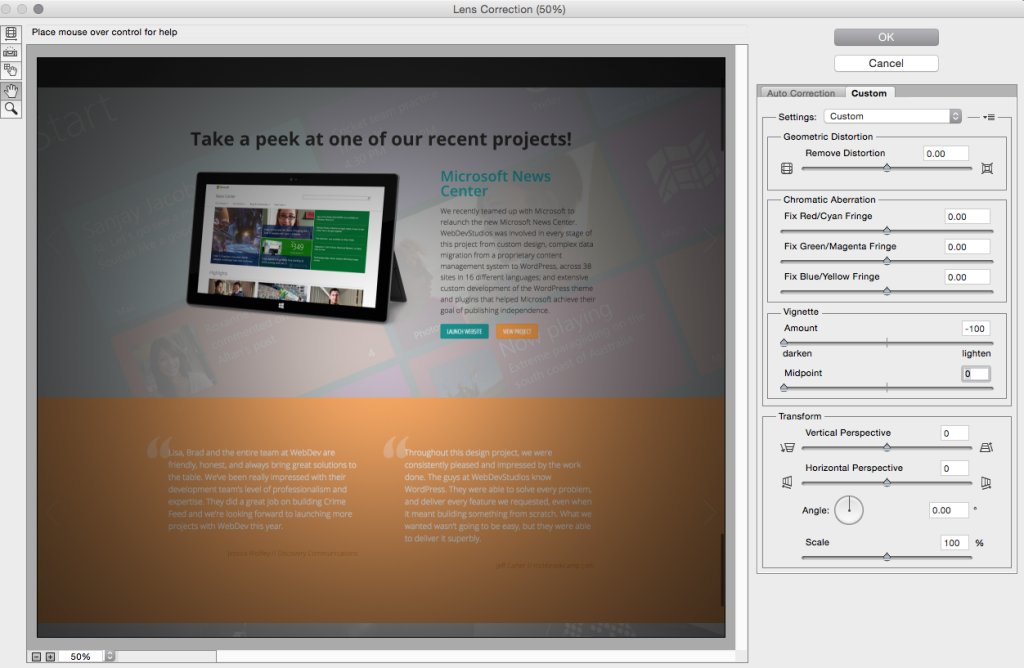
Total blindness
Want to feel embarrassed in a hurry? “View” your website with a screen reader and try to tab to your content. When I tested webdevstudios.com, I was frustrated while trying to skip all the social links, menu items, search bar, etc – for each page view!
To try this out, on OS X, press CMD + F5 to activate the screen reader. Then “Tab” through a web page.
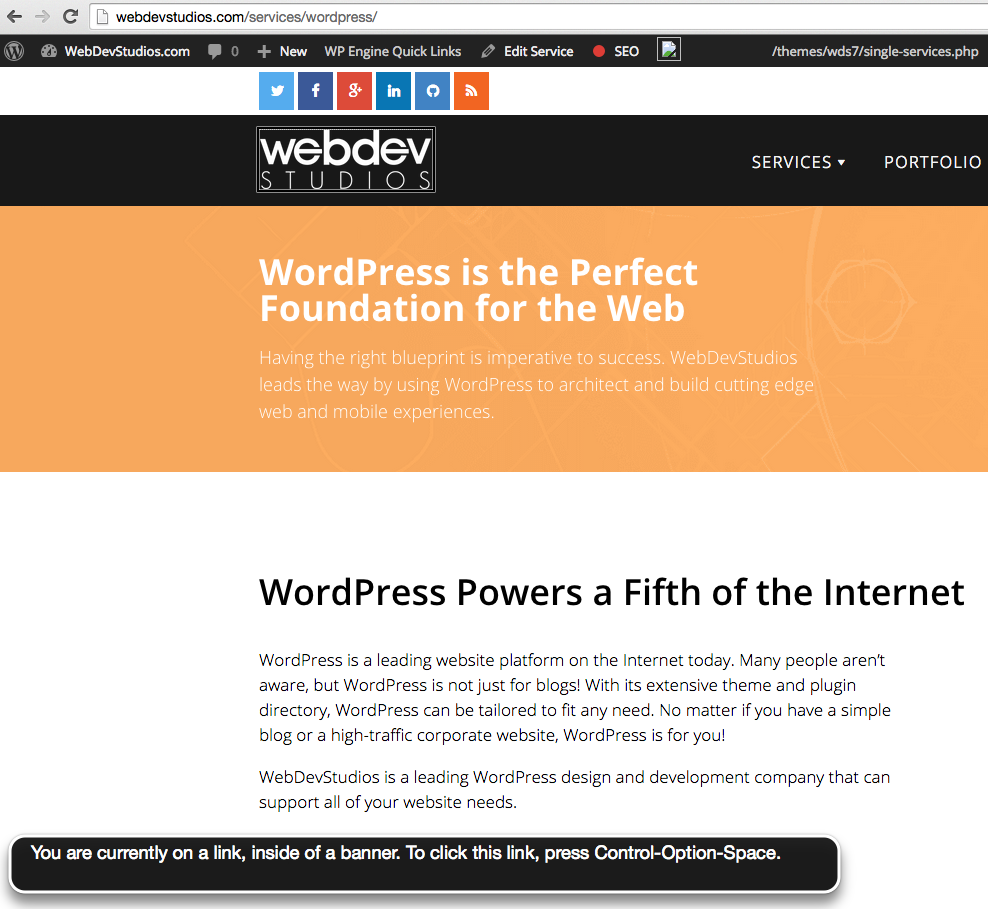
You could also simulate what a page might look like to a screen reader. Download the developer toolbar for Chrome, Firefox, or IE and trying disabling all styles:
Afterwards, you get a birds eye view of what webdevstudios.com migh look like to a screen reader:
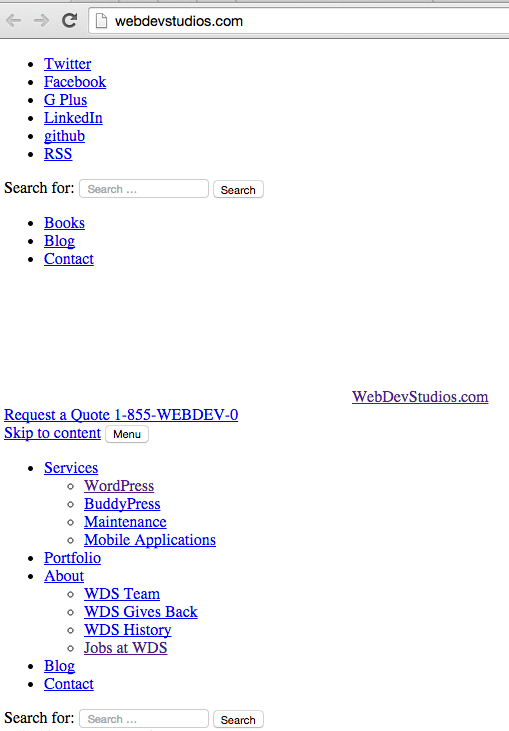
Now press “tab,” and continuing “tabbing” through the page. This is how a blind person might navigate your site.
Physical Impairment Techniques
Can you navigate your website with only a keyboard? Do your featured content sliders give users (without a mouse) enough time to navigate and take action? Are your form fields or hyperlinks large enough for someone who can still use a mouse (but has trouble) to click on/in? Are you putting your most important content into something that millions of people can’t see or operate?
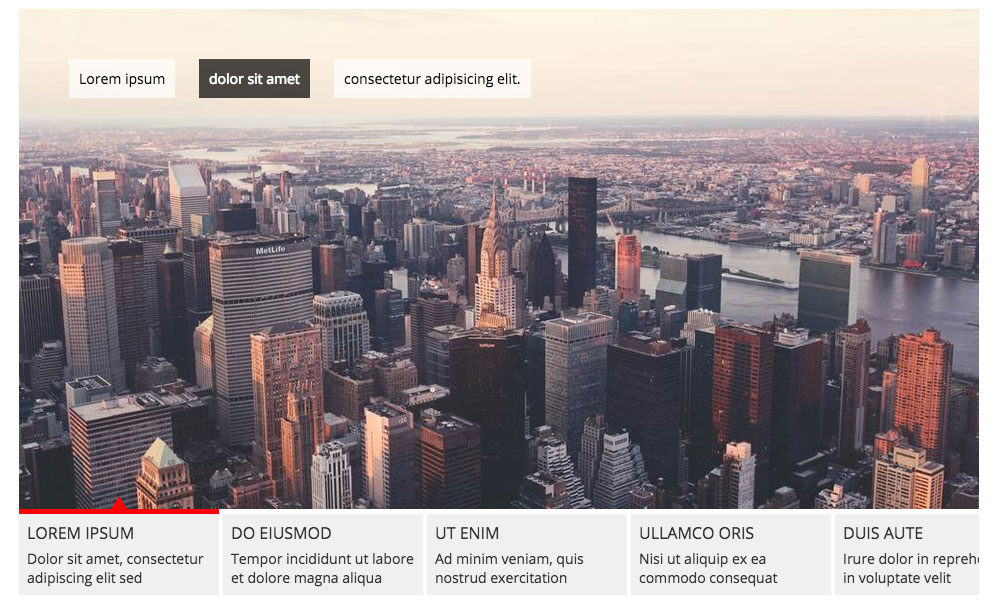
These are tough questions, which can easily be remedied by knowing how to use “tabindex” or adding padding to hyperlinks and form fields resulting in more “clickable area”. Tabindex is an attribute which aids in navigating a web page without a mouse. Tabindex tells the browser which order to follow when the user presses the “Tab” key. An example which would help navigating a form:
<a href="http://webdevstudios.com" tabindex="1">WebDevStudios</a> <form action="/" method="post"> <label for="name">First Name</label> <input type="text" id="name" tabindex="2"> <input type="submit" id="submitform" tabindex="3" value="Submit"> </form>
Hearing Impairment Techniques
Podcasts and Videos
If you host an audio or video podcast consider having it transcribed. You could ask a dedicated listener do “contribute to the show” by manually transcribing, maybe host your audio at YouTube or Vimeo and activate “closed captions,” or if your podcast is sponsored (or turn around time is important), consider a paid transcription service like CastingWords or Scribie.
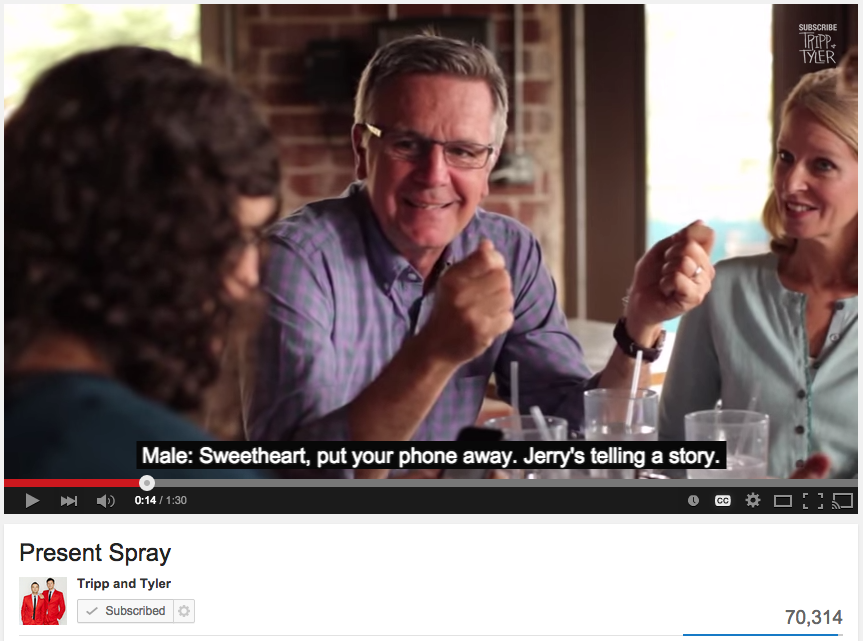
YouTube does a pretty good job at auto-magically figuring out both the word and the placement. While this is better than nothing, you may need to manually upload captions in a .scc format. You can learn more about closed caption file formats on YouTubes help page.
Get To Know ARIA
Combined with the semantic markup of HTML5, I believe ARIA is the easiest way to make your site more accessible. I really can’t provide a better description than the one provided by the W3C:
WAI-ARIA, the Accessible Rich Internet Applications Suite, defines a way to make Web content and Web applications more accessible to people with disabilities. It especially helps with dynamic content and advanced user interface controls developed with Ajax, HTML, JavaScript, and related technologies… With WAI-ARIA, developers can make advanced Web applications accessible and usable to people with disabilities. – W3C
In other words, WAI-ARIA is a tool to help us write code which can be interpreted by screen readers. There are three main components of ARIA are:
ARIA Roles
Roles tell a screen reader what the element is. For example, <nav role="navigation">
would indicate to the screen reader “This is a navigation element,” and would provide an easy way for the user to “jump” to it.
ARIA Properties
Properties define the properties or “meaning” of an element. <label aria-required="true">First Name</label>
would tell a user with a screen reader that this element is required.
ARIA States
States describe the current status of an element. <p aria-hidden="true">This content is hidden.</p>
would hide this from a screen reader, but not in a visual browser.
Learn more about ARIA
- Using ARIA in HTML
- ARIA visualized: A handy flow chart
- ARIA overview
- ARIA Roles
- HTML5 <hidden> and aria-hidden
- HTML5 vs ARIA redundancy
- ARIA Roles, Properties, and States
- Don’t Use Color For Web Forms, Use ARIA
I plan on writing an entire series of articles about ARIA, there’s that much to it.
Example Code Snippets
Screen Reader Text – The following is a great way to hide elements from a visual browser, yet allow them to be “seen” by screen reader:
/* Text meant only for screen readers. */ .screen-reader-text { clip: rect(1px, 1px, 1px, 1px); position: absolute !important; height: 1px; width: 1px; overflow: hidden; } .screen-reader-text&:hover, .screen-reader-text:active, .screen-reader-text:focus { background-color: #f1f1f1; border-radius: 3px; box-shadow: 0 0 2px 2px rgba(0, 0, 0, 0.6); clip: auto !important; color: #21759b; display: block; font-size: 14px; font-size: 1.4rem; font-weight: bold; height: auto; left: 5px; line-height: normal; padding: 15px 23px 14px; text-decoration: none; top: 5px; width: auto; z-index: 100000; /* Above WP toolbar. */ }
Tables – Using .screen-reader-text
and ARIA attributes to produce an accessible friendly table:
<table role="presentation"> <caption class="screen-reader-text">Duck Classifications</caption> <tr> <th scope="colgroup" colspan="4">Duck Name</th> </tr> <tr> <th scope="col">Puddle</th> <td>Mallard</td> <td>Pintail</td> <td>Teal</td> </tr> <tr> <th scope="col">Diving</th> <td>Canvasback</td> <td>Bufflehead</td> <td>Goldeneye</td> </tr> </table>
Forms – Make sure your forms include <label>
and tabindex
and ARIA labels
<form method="post" action="/" tabindex="2"> <label for="firstname" aria-label="First Name" tabindex="3">First Name:</label> <input type="text" required aria-required="true" name="firstname" id="firstname" tabindex="4"> <button type="submit" aria-label="Submit">Submit</button> </form>
Content Sliders – Using ARIA with featured content sliders:
<div role="slider" aria-valuenow="5" aria-valuemin="1" aria-valuemax="5" aria-valuetext="Friday"> <img src="images/tgif.jpg" alt="Someone celebrating Friday at work." /> </div>
Testing Tools
Screen Readers
- NVA Access (Windows, Free)
- JAWS (Windows, $1,000)
- VoiceOver (OS X, Free)
- Screen Reader Emulator (Firefox, Free)
Chrome’s Accessibility Developer Tools
- WAVE – Chrome extension
- Accessibility Developer Tools extension to aid in testing.
Automated Check/Validation
Total Validator is a free, downloadable app which will run a site (or a file) against a gamut of validation checks. Such as HTML, Accessibility, CSS, Spell Check, and can even check for broken links. Usage is straightforward: simply enter a URL and select your validation requirements… then click “Validate”.
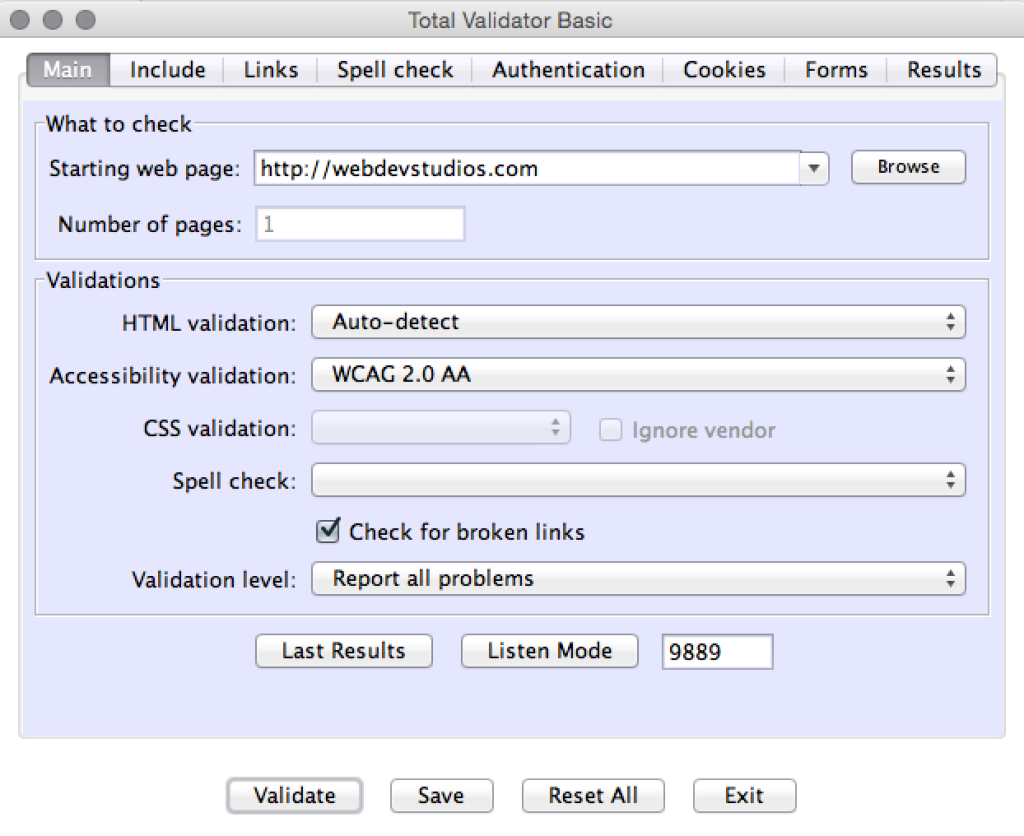
After a few moments, it will open the test results in a new tab:
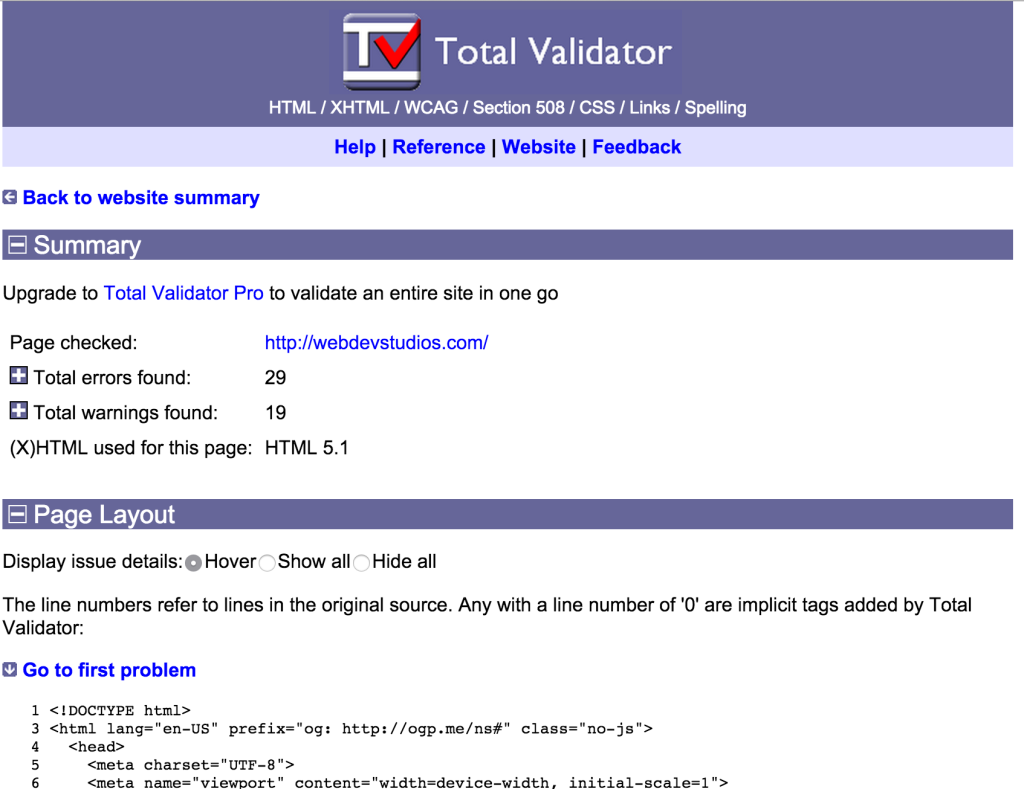
Total Validator also has browser extensions for Firefox and Chrome.
WordPress Plugins
WordPress Themes
Additional Resources
- Web Content Accessibility Guidelines (WCAG) Tutorials
- The a11y Project
- WordPress.tv
- Joe Dolson’s blog on accessibility
- Joseph Karr O’Conner’s blog on accessibility
- Theme Review Handbook on a11y
A challenge
I hope to have shown you that writing accessible code is not hard. If you’re willing to take some time to study WAI-ARIA (there’s a LOT of ARIA stuff!) you will be writing accessible code in no time. Before long, you’ll be in the habit of and writing it will become second nature.
Just to put our money where our mouth is:
Going forward at WebDevStudios: Our team of Leads has made a commitment to pushing out accessible code for clients. We plan to write it from the start of a project, and then test it during our QA process. I challenge other freelancers and agencies to do the same.
If you’re interested in getting involved, I would invite you to join the WordPress Accessibility Team or help make our new project boilerplate, wd_s, more accessible for the millions out there who don’t view the web in a traditional manner, because nobody should find it difficult to use the web.
Thanks for the article and for the tip about the WordPress Accessibility Team. I had no idea it existed. That’s really awesome about your team’s commitment to accessibility! Very important to take these things into consideration. They affect way more people than most developers would guess.
Accessibility for users with cognitive disabilities is also important. These articles have some good basic guidelines:
http://juicystudio.com/article/cognitive-impairment.php
http://webaim.org/articles/evaluatingcognitive/
I’m really glad to see that WDS is incorporating accessibility into all its projects going forward. Accessibility is a lot easier to achieve if you start from the beginning, and incorporating at the beginning can also save you and your clients a lot of money down the line.