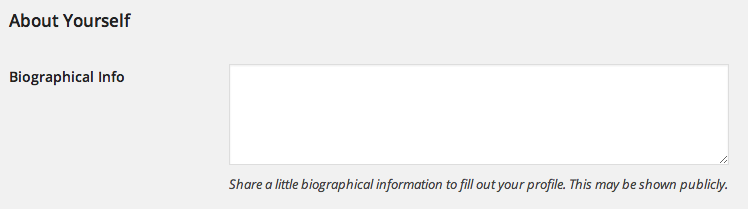
Wouldn’t it be great if the box above could be automatically filled by your Gravatar profile?
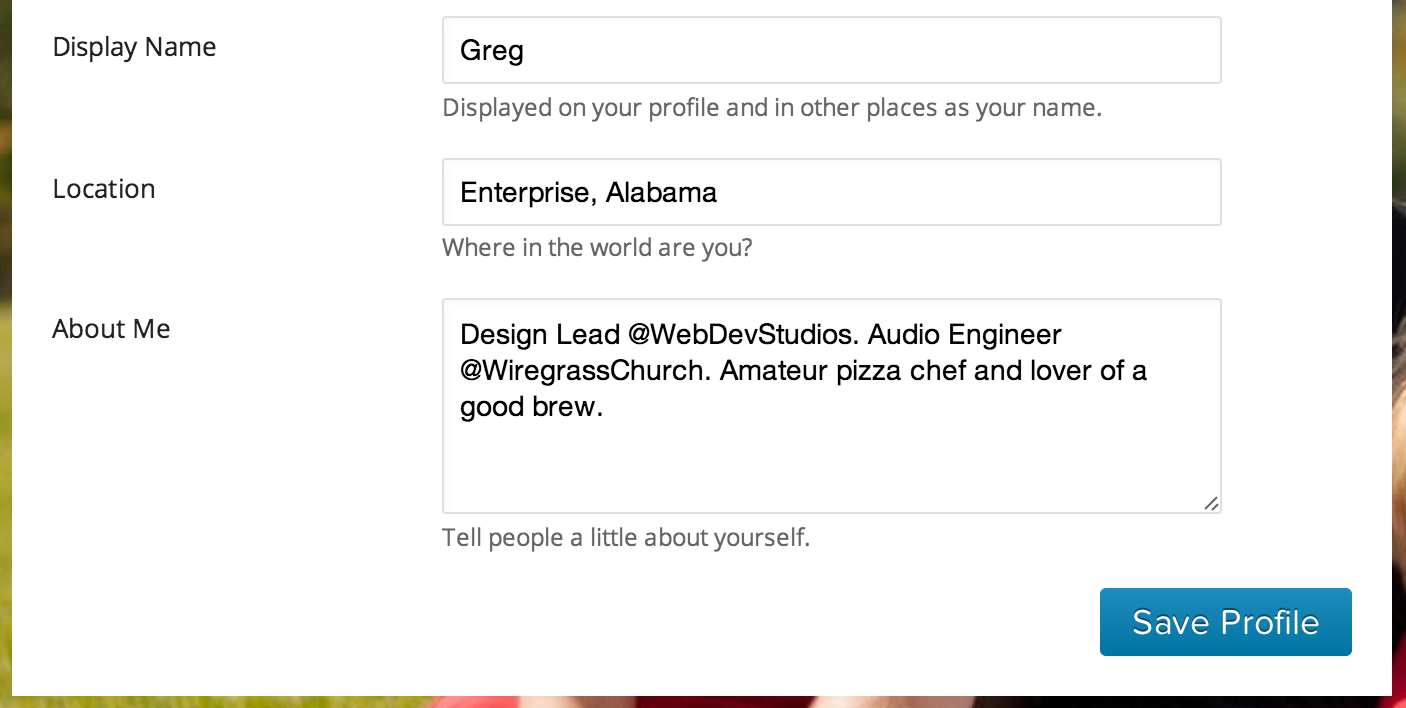
Tons of services auto-populate your info using Gravatar right? Why can’t my profile information (and social media info) just auto populate in WordPress too!? (NOTE: someone smarter than me should definitely work on getting this into WordPress core!)
Thankfully, it’s just a matter of tapping into the Gravatar API. Yup, there is a full fledged API with support for JSON, XML, PHP, VCF, and QR codes which makes it super easy to grab data.
A real world problem
Recently, a client was interested in adding an Author Box to each post. They run a large multi-site network (with over 100 authors) and asking the authors to fill out their bio on both Gravatar AND the WordPress dashboard (thus, repeating themselves) was out of the question.
The authors were asked to fill out their information once (on Gravatar) and I handled bringing in the data via the API.
A real solution
I built a function that gets the post author’s info using the Gravatar API.
<?php // DO NOT INCLUDE OPENING PHP TAG AND PLACE INTO FUNCTIONS.PHP /** * Get Author's Gravatar profile data. */ function custom_get_gravatar_profile() { // Get author data $author = strtolower( trim( get_the_author_meta( 'email' ) ) ); $author_ID = get_the_author_meta( 'ID' ); // Check for transient cache if ( false === ( $gravatar_profile = get_transient( 'gravatar_profile_' . $author_ID ) ) ) { // Check if author has a Gravatar $response = wp_remote_get( 'http://www.gravatar.com/' . md5( $author ) . '.php' ); // Did we recieve profile info? if ( ! 200 == wp_remote_retrieve_response_code( $response ) ) { return '<div id="message" class="error"><p>' . sprintf( __( 'This user does not have a Gravatar! Why not <a href="%s">set up a profile</a>?', 'textdomain' ), 'http://en.gravatar.com/' ) . '</p></div>'; } // Parsing profile data from Gravatar response $body = unserialize( wp_remote_retrieve_body( $response ) ); // Is our data in an array? if ( ! is_array( $body ) ) { return '<div id="message" class="error"><p>' . __( 'There was an error extracting the profile from Gravatar's API. Please try again later.', 'textdomain' ) . '</p></div>'; } // Shift the profile data, which is two levels deep into an array $gravatar_profile = current( current( $body ) ); // Place profile in a transient, expire after 12 hours set_transient( 'gravatar_profile_' . $author_ID, $gravatar_profile, 12 * HOUR_IN_SECONDS ); } // Return the profile return $gravatar_profile; }
This above function returns an array of data:
Array ( [id] => 3631430 [hash] => 28af3e39c0a1fe4c31367c7e9a8bcac3 [requestHash] => 28af3e39c0a1fe4c31367c7e9a8bcac3 [profileUrl] => http://gravatar.com/gregrickaby [preferredUsername] => gregrickaby [thumbnailUrl] => http://1.gravatar.com/avatar/28af3e39c0a1fe4c31367c7e9a8bcac3 [photos] => Array ( [0] => Array ( [value] => http://1.gravatar.com/avatar/28af3e39c0a1fe4c31367c7e9a8bcac3 [type] => thumbnail ) ) [currency] => Array ( ) [profileBackground] => Array ( [color] => # [url] => https://secure.gravatar.com/bg/3631430/d3a13611c357b6cfe4945d329ea2f175 ) [name] => Array ( [givenName] => Greg [familyName] => Rickaby [formatted] => Gregory James Rickaby ) [displayName] => Greg [aboutMe] => Design Lead @WebDevStudios. Audio Engineer @WiregrassChurch. Amateur pizza chef and lover of a good brew. [currentLocation] => Enterprise, Alabama [accounts] => Array ( [0] => Array ( [domain] => facebook.com [display] => gregrickaby [url] => http://www.facebook.com/gregrickaby [username] => gregrickaby [verified] => true [shortname] => facebook ) [1] => Array ( [domain] => profiles.google.com [display] => profiles.google.com [url] => http://profiles.google.com/115960795578445596136 [userid] => 115960795578445596136 [verified] => true [shortname] => google ) [2] => Array ( [domain] => twitter.com [display] => @GregRickaby [url] => http://twitter.com/GregRickaby [username] => GregRickaby [verified] => true [shortname] => twitter ) [3] => Array ( [domain] => youtube.com [display] => gregrickaby [url] => http://www.youtube.com/user/gregrickaby [username] => gregrickaby [verified] => true [shortname] => youtube ) ) [urls] => Array ( [0] => Array ( [value] => http://gregrickaby.com [title] => My Blog ) ) )
This particular client is using Genesis, so I hooked into the Author Box filter, looped through the array, and extracted the data:
<?php // DO NOT INCLUDE OPENING PHP TAG AND PLACE INTO FUNCTIONS.PHP add_filter( 'genesis_author_box', 'custom_author_box' ); /** * Author box */ function custom_author_box() { // Get author data $author = custom_get_gravatar_profile(); // Set variables $author_avatar = $author['thumbnailUrl']; $author_first = $author['name']['givenName']; $author_last = $author['name']['familyName']; $author_full_name = $author_first . ' ' . $author_last; $author_bio = $author['aboutMe']; ?> <div class="author-box"> <img src="<?php echo esc_url( $author_avatar ); ?>" class="avatar author-avatar alignleft" alt="<?php echo esc_html( $author_full_name ); ?>" title="<?php esc_html( $author_full_name ); ?>" height="70" width="70" /> <p class="author-name"><strong><?php _e( 'About', 'realtor' ); ?> <?php echo esc_html( $author_full_name ); ?></strong></p> <p class="author-bio"><?php echo esc_html( $author_bio ); ?></p> <div class="author-box"> <img src="<?php echo esc_url( $author_avatar ); ?>" class="avatar author-avatar alignleft" alt="<?php echo esc_html( $author_full_name ); ?>" title="<?php esc_html( $author_full_name ); ?>" height="70" width="70" /> <p class="author-name"><strong><?php _e( 'About', 'realtor' ); ?> <?php echo esc_html( $author_full_name ); ?></strong></p> <p class="author-bio"><?php echo esc_html( $author_bio ); ?></p> <?php if ( $author['accounts'] ) : ?> <ul class="author-socials"> <?php foreach ( $author['accounts'] as $key => $account ) : ?> <li class="author-social"><a href="<?php echo esc_url( $account['url'] ); ?>"><i class="genericon genericon-<?php echo esc_html( $account['shortname'] ); ?>"></i></a></li> <?php endforeach; ?> </ul> <?php endif; ?> </div><!-- .author-box --> </div><!-- .authorbox --> <?php }
The final product, an author box with profile information from Gravatar’s API:
Pretty easy, huh? Now stop repeating yourself, and start using the Gravatar API!
(The above code can be forked from this Github Gist)
Great article! One suggestion I have is to pull json since typically json_decode is faster than unserialize. It’s also much safer to decode json in the crazy event someone hacks Gravatar and pushes malicious code out.