The amount of thought and time that goes into designing a universally accepted icon is amazing. Choosing when to utilize icons to represent interfacing elements is paramount to whether users will understand, and seamlessly navigate areas of a website.
Previously, website icons consisted of small images, and sometimes image sprites. Creating and maintaining these images was cumbersome, and integration tedious at times.
Recently, icon fonts have paved the way for expedient delivery of icons. Libraries of font icons have become prevalent, one of which is Font Awesome.
Let’s get started with integrating Font Awesome icons with bbPress forums, but first I would like to point out this bbPress Trac ticket that created the filter necessary to add this functionality. This may be a helpful reference: “New Filters for Topic/Reply Admin Links.”
First we must enqueue the Font Awesome library script in our theme. Although, you could use a plugin: Font Awesome Icons, but we’re going to be smart about it since we’re going to be utilizing Font Awesome in other areas of our site, and keep it in our theme.
wp_enqueue
to the rescue!
$cdn_url = '//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css'; wp_register_style( 'font-awesome', $cdn_url ); wp_enqueue_style( 'font-awesome' );
Drop that snippet in your theme’s functions.php
file, and now we have Font Awesome loading on every page. (Of course, if you would like to limit loading to only the necessary pages you could use one of the many handy-dandy conditionals.)
We’re going to start by adding an icon to bbPress’s default ‘Edit’ link for a topic.

We’ll hook into the bbp_topic_admin_links
filter and add our icon font, like so (again, functions.php
):
/** * bbPress - replace topic edit links with Font Awesome icons */ function bbp_topic_icon_admin_link( $links ) { $links['edit'] = bbp_get_topic_edit_link( array( 'edit_text' => '<i class="fa fa-edit" title="'. __( 'Edit topic', 'wds-tutorial-example' ) .'"></i>'. __( 'Edit', 'wds-tutorial-example' ), ) ); return $links; } add_filter( 'bbp_topic_admin_links', 'bbp_topic_icon_admin_link' );
That gives us this:

Now we’ll want to do the same for the reply to topic area by tying into the bbp_reply_admin_links
filter so it’ll look like this:
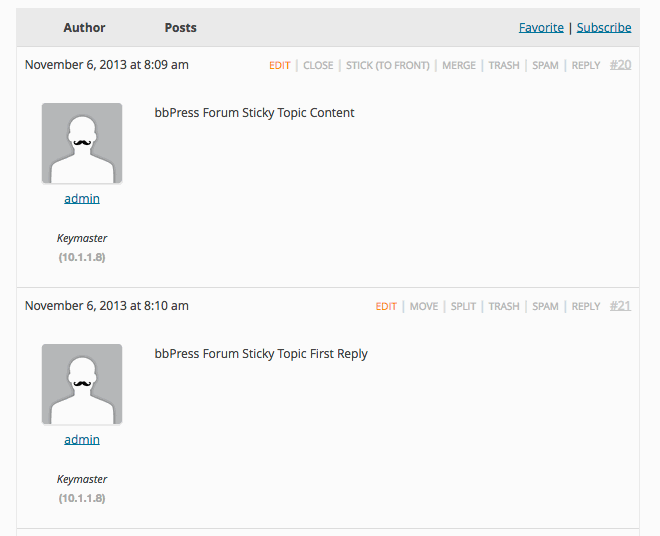
We can hook into reply links with this (functions.php
):
/** * bbPress - replace reply links with Font Awesome icons */ function bbp_reply_icon_admin_links( $links ) { $links['edit'] = bbp_get_reply_edit_link( array( 'edit_text' => '<i class="fa fa-edit" title="'. __( 'Edit topic', 'wds-tutorial-example' ) .'"></i>'. __( 'Edit', 'wds-tutorial-example' ), ) ); return $links; } add_filter( 'bbp_reply_admin_links', 'bbp_reply_icon_admin_links' );
Of course, you can add an icon to any one of those links, and even replace the link altogether, like:
<?php /** * bbPress - replace topic edit links with Font Awesome icons */ function bbp_topic_icon_admin_link( $links ) { $links['edit'] = bbp_get_topic_edit_link( array( 'edit_text' => '<i class="fa fa-edit" title="'. __( 'Edit Topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Edit', 'wds-tutorial-example' ) .'</span>' ) ); $links['close'] = bbp_get_topic_close_link( array( 'close_text' => '<i class="fa fa-toggle-on" title="'. __( 'Close topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Close', 'wds-tutorial-example' ) .'</span>', 'open_text' => '<i class="fa fa-toggle-off" title="'. __( 'Open topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Open', 'wds-tutorial-example' ) .'</span>', ) ); $links['stick'] = bbp_get_topic_stick_link( array( 'stick_text' => '<i class="fa fa-bookmark" title="'. __( 'Stick topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Stick', 'wds-tutorial-example' ) .'</span>', 'unstick_text' => '<i class="fa fa-bookmark-o" title="'. __( 'Unstick topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Unstick', 'wds-tutorial-example' ) .'</span>', 'super_text' => '<i class="fa fa-asterisk" title="'. __( 'Stick to front', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Stick to front', 'wds-tutorial-example' ) .'</span>', ) ); $links['merge'] = bbp_get_topic_merge_link( array( 'merge_text' => '<i class="fa fa-exchange" title="'. __( 'Merge topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Merge', 'wds-tutorial-example' ) .'</span>' ) ); $links['trash'] = bbp_get_topic_trash_link( array( 'trash_text' => '<i class="fa fa-trash" title="'. __( 'Trash topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">Trash</span>', 'restore_text' => '<i class="fa fa-restore" title="'. __( 'Restore topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Restore', 'wds-tutorial-example' ) .'</span>', 'delete_text' => '<i class="fa fa-times" title="'. __( 'Delete topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Delete', 'wds-tutorial-example' ) .'</span>', ) ); $links['spam'] = bbp_get_topic_spam_link( array( 'spam_text' => '<i class="fa fa-exclamation" title="'. __( 'Mark as spam', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Spam', 'wds-tutorial-example' ) .'</span>', 'unspam_text' => '<i class="fa fa-exclamation-circle" title="'. __( 'Unmark as spam', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Unspam', 'wds-tutorial-example' ) .'</span>', ) ); $links['reply'] = bbp_get_topic_reply_link( array( 'reply_text' => '<i class="fa fa-reply" title="'. __( 'Reply', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Reply', 'wds-tutorial-example' ) .'</span>' ) ); return $links; } add_filter( 'bbp_topic_admin_links', 'bbp_topic_icon_admin_link' ); /** * bbPress - replace reply links with Font Awesome icons */ function bbp_reply_icon_admin_links( $links ) { $links['edit'] = bbp_get_reply_edit_link( array( 'edit_text' => '<i class="fa fa-edit" title="'. __( 'Edit topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Edit', 'wds-tutorial-example' ) .'</span>' ) ); $links['move'] = bbp_get_reply_move_link( array( 'split_text' => '<i class="fa fa-arrow-circle-right" title="'. __( 'Move topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Move', 'wds-tutorial-example' ) .'</span>' ) ); $links['split'] = bbp_get_topic_split_link( array( 'split_text' => '<i class="fa fa-code-fork" title="'. __( 'Split topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Split', 'wds-tutorial-example' ) .'</span>' ) ); $links['trash'] = bbp_get_reply_trash_link( array( 'trash_text' => '<i class="fa fa-trash" title="'. __( 'Trash topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Trash', 'wds-tutorial-example' ) .'</span>', 'restore_text' => '<i class="fa fa-restore" title="'. __( 'Restore topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Restore', 'wds-tutorial-example' ) .'</span>', 'delete_text' => '<i class="fa fa-times" title="'. __( 'Delete topic', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Delete', 'wds-tutorial-example' ) .'</span>', ) ); $links['spam'] = bbp_get_reply_spam_link( array( 'spam_text' => '<i class="fa fa-exclamation" title="'. __( 'Mark as spam', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Spam', 'wds-tutorial-example' ) .'</span>', 'unspam_text' => '<i class="fa fa-exclamation-circle" title="'. __( 'Unmark as spam', 'wds-tutorial-example' ) .'"></i><span class="screen-reader-text">'. __( 'Unspam', 'wds-tutorial-example' ) .'</span>', ) ); return $links; } add_filter( 'bbp_reply_admin_links', 'bbp_reply_icon_admin_links' );
Which will give you this:
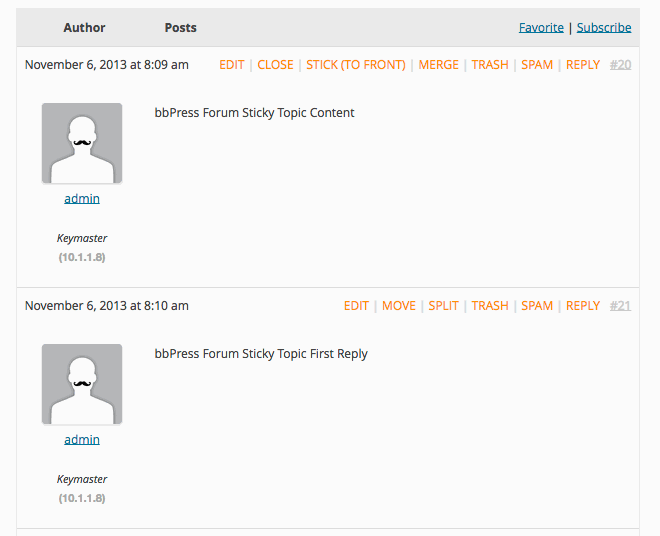
But that is just crazy TOWN! Would you know what each icon represented if you were a new user trying to interact with that forum? Me neither. So be careful when and where you use such a recipe (for disaster?).
Integrating icons into modern web interfaces is a continuing challenge that can be fun if used properly. Have fun!
Very clever !
It has been a while since I last used BBP, but how about enabling tooltips upon hovering over icons ? In this way newcomers can know what each icon represented
Hi Jose! Are we talking about some javascript tooltips? There already is
title
attribute.